Using the Share Dialog¶
If you currently have a share function in your app you are most likely using the default UIActivityViewController provided by the iOS platform.
The Loopy™ Share Dialog leverages UIActivityViewController but provides important callbacks to allow more sophisticated social analytics.
To implement the Loopy™ Share Dialog, simply use the STAPIClient and STShareActivityUI classes, along with the STActivity protocol, to create a trackable URL and display it using the default share dialog.
As with the iOS default UIActivityViewController, STShareActivityUI offers Facebook and Twitter sharing, as well as the ability to add additional UIActivity implementations you may have representing additional social networks or other sharing services.
If you don’t already have one, add a button to your UIView layout (either in XIB file, pictured below, or programatically):
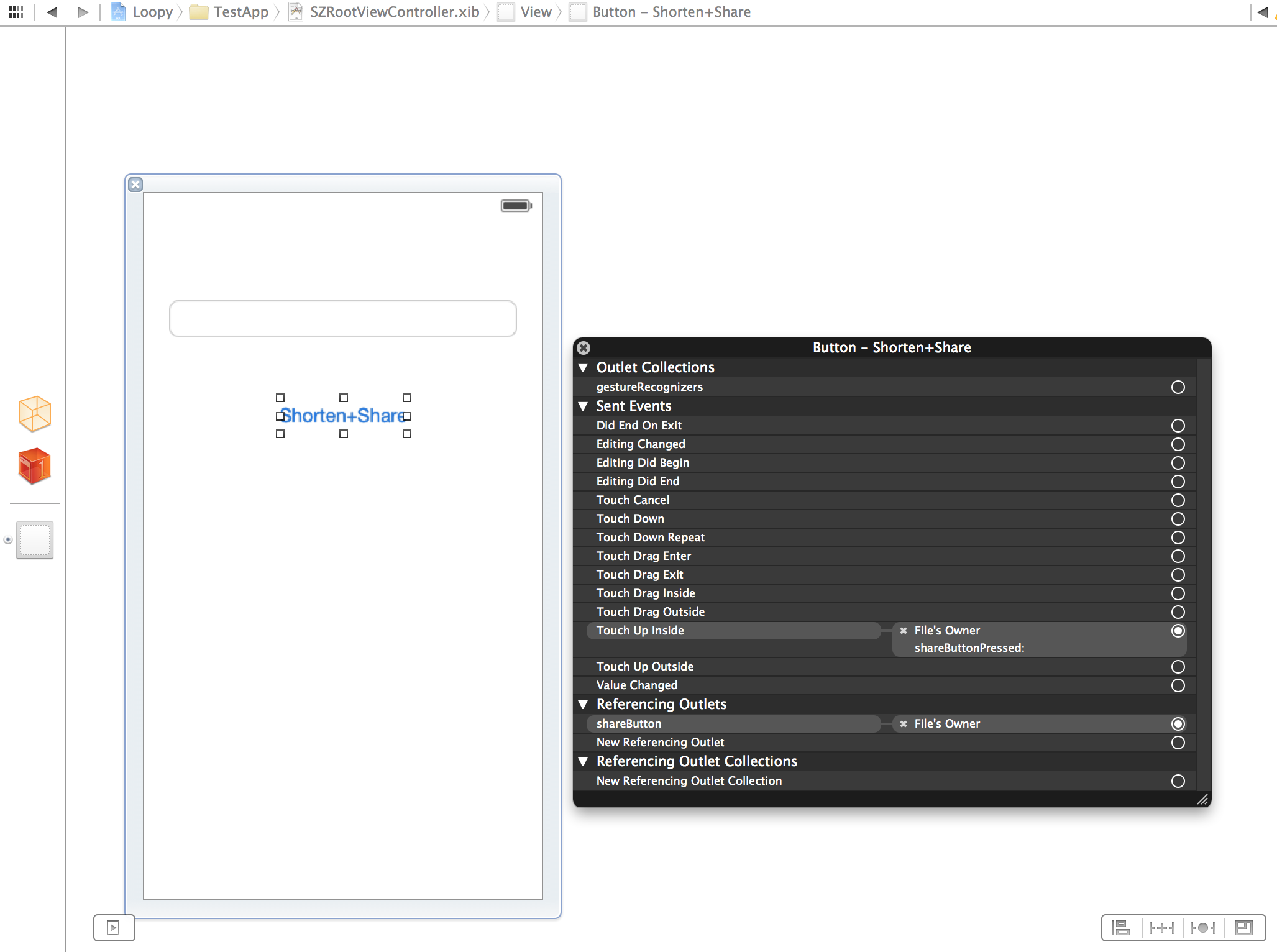
Add an event handler for when the button is pressed (in this example, the Touch Up Inside event handled by an IBAction).
Note
- If you have event-handling code that needs to execute after certain share actions, see the examples in the snippet using NSNotificationCenter
//Returns a shortened URL then launches the Share Dialog
- (IBAction)shortlinkButtonPressed:(id)sender {
//init this and begin its session elsewhere
STAPIClient *apiClient;
//replace with your own view controller
UIViewController *myViewController = [[UIViewController alloc] init];
//EVENT HANDLING FOR SHARE ACTIVITIES (optional):
//called when user taps on one of the social network or other share-type buttons
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(handleShareDidBegin:)
name:LoopyShareDidBegin
object:nil];
//called when user taps "Cancel" in the dialog to share to a particular social network
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(handleShareDidCancel:)
name:LoopyShareDidCancel
object:nil];
//called when item was shared successfully to a particular social network
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(handleShareDidComplete:)
name:LoopyShareDidComplete
object:nil];
//called when item was shared successfully to another medium (e.g. e-mail, SMS)
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(handleActivityDidBegin:)
name:LoopyActivityDidComplete
object:nil];
//called when user taps "Cancel" from the activity dialog WITHOUT choosing a social network or other share type
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(handleActivityDidCancel:)
name:LoopyActivityDidCancel
object:nil];
//called when share was successfully recorded by Loopy
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(handleRecordShareDidSucceed:)
name:LoopyRecordShareDidSucceed
object:nil];
//called when share FAILED to be recorded by Loopy
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(handleRecordShareDidFail:)
name:LoopyRecordShareDidFail
object:nil];
//init share activity UI to build share dialog
STShareActivityUI *share = [[STShareActivityUI alloc] initWithParent:myViewController apiClient:apiClient];
NSArray *tags = [NSArray arrayWithObjects:@"tag1", @"tag2", nil];
STShortlink *shortlinkObj = [apiClient shortlinkWithURL:@"www.UrlToShorten.com" title:nil meta:nil tags:tags];
[apiClient shortlink:shortlinkObj
success:^(AFHTTPRequestOperation *operation, id responseObject) {
//use the shortlink in the activity dialog
NSDictionary *responseDict = (NSDictionary *)responseObject;
NSString *shortlink = (NSString *)[responseDict valueForKey:@"shortlink"];
NSArray *activityItems = @[shortlink];
NSArray *activities = [share getDefaultActivities:activityItems];
UIActivityViewController * activityController = [share newActivityViewController:activityItems
withActivities:activities];
[share showActivityViewDialog:activityController completion:nil];
}
failure:^(AFHTTPRequestOperation *operation, NSError *error) {
//any failure handling
}];
}
To add additional UIActivity classes to the Loopy™ Share Dialog, create your custom UIActivities (or modify your existing ones) to conform to the STActivity protocol; your header and implementation files should contain the following:
#import <UIKit/UIKit.h>
#import "STActivity.h"
@interface MyCustomActivity : UIActivity<STActivity>
@property (nonatomic, strong) NSArray *shareItems;
@end
@implementation MyCustomActivity
@synthesize shareItems;
//Standard UIActivity implementation
- (NSString *)activityTitle {
return @"MyCustomActivity";
}
//Standard UIActivity implementation
- (NSString *)activityType {
return @"MyActivityType";
}
//Standard UIActivity implementation
- (UIImage *)activityImage {
UIImage *image = nil;
//return an image that's appropriately sized for all devices and OSes:
//iOS 7 iPhone and non-iOS7 iPads: 60x60
//non-iOS7 iPhone: 43 x 43
//iOS 7 iPad: 76 x 76
return image;
}
//Any final checking of activities or sharing service passed in can be done here
- (BOOL)canPerformWithActivityItems:(NSArray *)activityItems {
return YES;
}
//Notification of intent to share
//Set shareItems and post notification here
- (void)prepareWithActivityItems:(NSArray *)activityItems {
self.shareItems = activityItems;
[[NSNotificationCenter defaultCenter] postNotificationName:LoopyShareDidBegin object:self];
}
@end
Include the custom activity in the NSArray passed in to STShare’s UIActivityViewController, as follows:
//This is an example of an activity controller with custom UIActivities added in
- (UIActivityViewController *)activityControllerWithCustomActivities:(NSArray *)activityItems {
// STShare *share = [[STShare alloc] initWithParent:self];
// NSArray *defaultActivities = [share getDefaultActivities:activityItems];
//
// //add in any custom activities
// NSMutableArray *allActivities = [NSMutableArray arrayWithArray:defaultActivities];
// MyCustomActivity *customActivity = [MyCustomActivity initWithActivityItems:activityItems];
// [allActivities addObject:customActivity];
//
// UIActivityViewController *activityController = [share newActivityViewController:activityItems
// withActivities:allActivities];
//
// return activityController;
return nil;
}