Custom Like Buttons¶
One of the most commonly used social features is a simple “like” button. Socialize provides a way to quickly and easily create a customized like button that you can include in your app with minimal effort.
The following step-by-step guide will show you how to include a basic like button with custom images and text.
How-To Guide¶
Step 1 - Setup Socialize¶
If you haven’t already, make sure you have successfully completed the Getting Started process
Step 2 - Create a Button State Drawable¶
If you want your like button to match the look and feel of your app you can do this by creating a StateListDrawable as an XML configuration saved to res/drawable
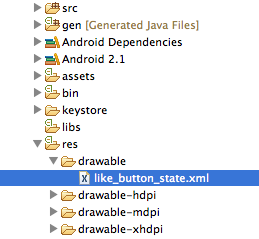
<!--
Create a state list drawable to represent the button states then
add to res/drawable/like_button_state.xml
-->
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:drawable="@drawable/like"
android:state_checked="true"/>
<item
android:drawable="@drawable/unlike"
android:state_checked="false"/>
</selector>
This example is using two images from our demo app called “like” and “unlike”
![]() |
![]() |
Step 3 - Create a Button¶
The Socialize Like Button behavior can be applied to any type of CompoundButton. This includes CheckBox, RadioButton, ToggleButton and Switch (API 11)
To turn one of these button types into a Socialize Like Button, first define a button in your layout. The following example uses a Checkbox:
<!-- Set the android:button attribute to your state list drawable -->
<CheckBox
android:id="@+id/btnCustomCheckBoxLike"
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:layout_gravity="left|center_vertical"
android:button="@drawable/like_button_state"/>
Step 4 - Transform the Button¶
The final step in the process is to transform your button into a Socialize Like Button.
In the onCreate method of your Activity simply call the makeLikeButton method on the LikeUtils instance.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Define/obtain your entity
Entity entity = Entity.newInstance("http://getsocialize.com", "Socialize");
// Get a reference to the button you want to transform
// This can be any type of CompoundButton (CheckBox, RadioButton, Switch, ToggleButton)
CheckBox btnCustomCheckBoxLike = (CheckBox) findViewById(R.id.btnCustomCheckBoxLike);
// Make the button a socialize like button!
LikeUtils.makeLikeButton(this, btnCustomCheckBoxLike, entity, new LikeButtonListener() {
@Override
public void onClick(CompoundButton button) {
// You can use this callback to set any loading text or display a progress dialog
button.setText("--");
}
@Override
public void onCheckedChanged(CompoundButton button, boolean isChecked) {
// The like was posted successfully, change the button to reflect the change
if(isChecked) {
button.setText("Unlike");
}
else {
button.setText("Like");
}
}
@Override
public void onError(CompoundButton button, Exception error) {
// An error occurred posting the like, we need to return the button to its original state
Log.e("Socialize", "Error on like button", error);
if(button.isChecked()) {
button.setText("Unlike");
}
else {
button.setText("Like");
}
}
});
}
This will result in a completely custom Socialize Like Button!
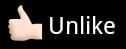