Comments¶
UI Elements¶
Comments List¶
The standard Socialize Comment List UI is included with the Socialize Action Bar however if you wanted to create your own ActionBar or simply want to launch the Comment List from elsewhere in your app this can simply be done with a few lines of code
- (void)showCommentsList {
SZEntity *entity = [SZEntity entityWithKey:@"some_key_or_url" name:@"Something"];
[SZCommentUtils showCommentsListWithViewController:self entity:entity completion:nil];
}
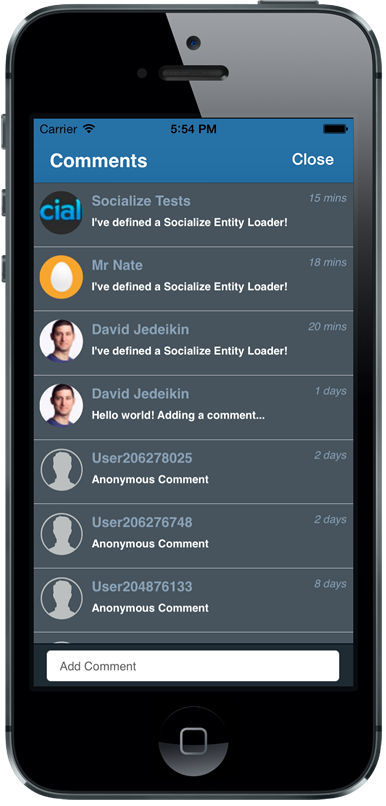
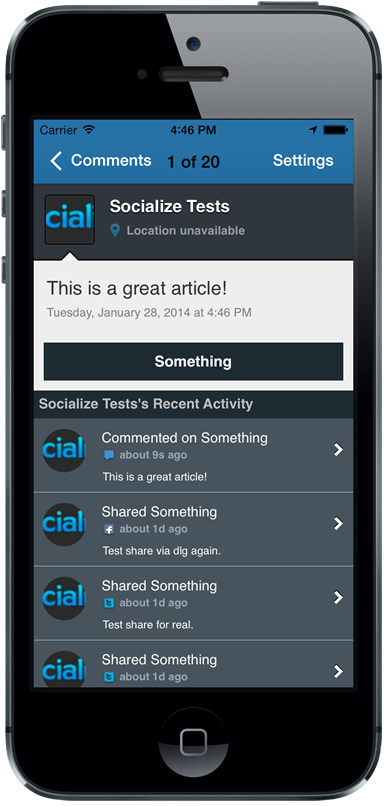
If you need to, you can directly instantiate the comments list and manage its lifecycle on your own.
Note
You could use comments options to prefill comment with custom message.
- (void)manuallyShowCommentsList {
SZCommentOptions* options = [SZCommentUtils userCommentOptions];
options.text = @"Hello world";
SZEntity *entity = [SZEntity entityWithKey:@"key" name:@"name"];
SZCommentsListViewController *comments = [[SZCommentsListViewController alloc] initWithEntity:entity];
comments.completionBlock = ^{
// Dismiss however you want here
[self dismissViewControllerAnimated:NO completion:nil];
};
comments.commentOptions = options;
// Present however you want here
[self presentViewController:comments animated:NO completion:nil];
}
Comment Composer¶
Although the comments list opens a composer, a standalone comment composition controller is also provided:
- (void)showCommentComposer {
SZEntity *entity = [SZEntity entityWithKey:@"some_key_or_url" name:@"Something"];
[SZCommentUtils showCommentComposerWithViewController:self entity:entity completion:^(id<SZComment> comment) {
NSLog(@"Created comment: %@", [comment text]);
} cancellation:^{
NSLog(@"Cancelled comment create");
}];
}
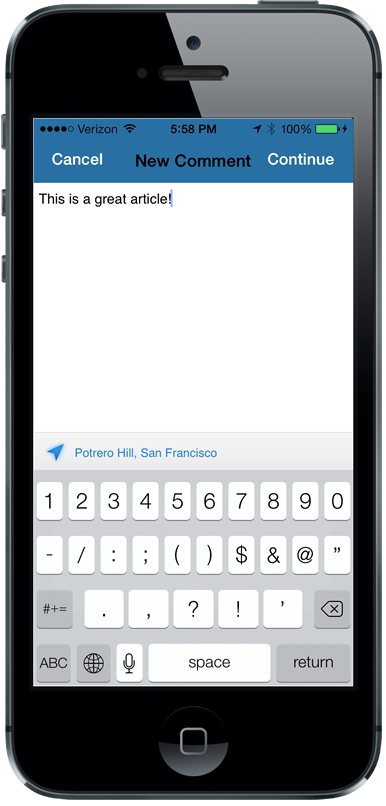
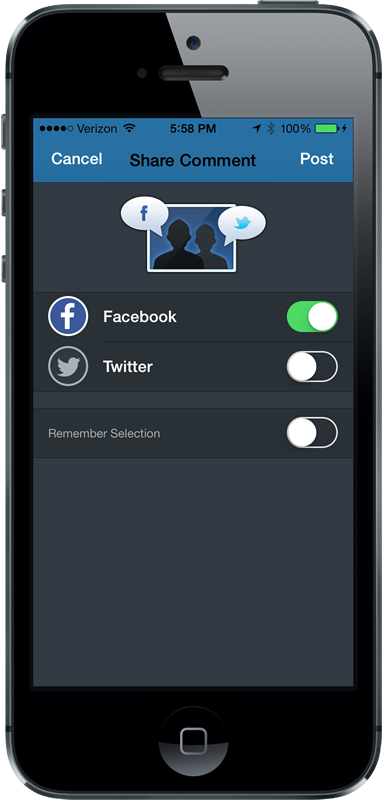
You can also directly instantiate the comment composer
Note
You could use comments options to prefill comment with custom message.
- (void)manuallyShowCommentComposer {
SZEntity *entity = [SZEntity entityWithKey:@"key" name:@"name"];
SZComposeCommentViewController *composer = [[SZComposeCommentViewController alloc] initWithEntity:entity];
composer.completionBlock = ^(id<SZComment> comment) {
NSLog(@"Created comment: %@", [comment text]);
// Dismiss however you want here
[self dismissViewControllerAnimated:NO completion:nil];
};
// Present however you want here
[self presentViewController:composer animated:NO completion:nil];
}
Working with comments¶
Creating Comments, specify networks¶
To create a comment programmatically you simply call the addComment method on CommentUtils
Similar to shares, you can specify how the comment is to be propagated to 3rd party networks
- (void)addComment {
SZEntity *entity = [SZEntity entityWithKey:@"some_key_or_url" name:@"Something"];
SZCommentOptions *options = [SZCommentUtils userCommentOptions];
options.willAttemptPostingToSocialNetworkBlock = ^(SZSocialNetwork network, SZSocialNetworkPostData *postData) {
if (network == SZSocialNetworkFacebook) {
[postData.params setObject:@"Custom message" forKey:@"message"];
} else if (network == SZSocialNetworkTwitter) {
[postData.params setObject:@"Custom status" forKey:@"status"];
}
};
[SZCommentUtils addCommentWithEntity:entity text:@"A Comment!" options:options networks:SZAvailableSocialNetworks() success:^(id<SZComment> comment) {
NSLog(@"Created comment: %@", [comment text]);
} failure:^(NSError *error) {
NSLog(@"Failed creating comment: %@", [error localizedDescription]);
}];
}
Create comment, prompt for networks¶
If you’d to create a comment and specify text but not networks, you can do so. You might use this to build your own comment composer but still show the Socialize network selection dialog.
- (void)addCommentPromptForNetworks {
SZEntity *entity = [SZEntity entityWithKey:@"some_key_or_url" name:@"Something"];
SZCommentOptions *options = [SZCommentUtils userCommentOptions];
[SZCommentUtils addCommentWithViewController:self entity:entity text:@"A comment!" options:options success:^(id<SZComment> comment) {
NSLog(@"Created comment: %@", [comment text]);
} failure:^(NSError *error) {
NSLog(@"Failed creating comment: %@", [error localizedDescription]);
}];
}
Retrieving Comments¶
You can retrieve existing comments by User, Entity or directly using an ID
List comments by User
- (void)listCommentsByUser {
[SZCommentUtils getCommentsByUser:nil first:nil last:nil success:^(NSArray *comments) {
NSLog(@"Fetched comments: %@", comments);
} failure:^(NSError *error) {
NSLog(@"Failed: %@", [error localizedDescription]);
}];
}
List comments by Entity
- (void)listCommentsByEntity {
SZEntity *entity = [SZEntity entityWithKey:@"some_key_or_url" name:@"Something"];
[SZCommentUtils getCommentsByEntity:entity success:^(NSArray *comments) {
NSLog(@"Fetched comments: %@", comments);
} failure:^(NSError *error) {
NSLog(@"Failed: %@", [error localizedDescription]);
}];
}
List comments by User on a specific entity
- (void)listCommentsByUserAndEntity {
SZEntity *entity = [SZEntity entityWithKey:@"some_key_or_url" name:@"Something"];
[SZCommentUtils getCommentsByUser:USER_ADDR_NULL entity:entity first:nil last:nil success:^(NSArray *comments) {
NSLog(@"Fetched comments: %@", comments);
} failure:^(NSError *error) {
NSLog(@"Failed: %@", [error localizedDescription]);
}];
}
List comments by ID
- (void)listCommentsByIds {
// I've stashed these ids somewhere
NSArray *ids = [NSArray arrayWithObjects:[NSNumber numberWithInteger:1], [NSNumber numberWithInteger:1], nil];
[SZCommentUtils getCommentsWithIds:ids success:^(NSArray *comments) {
NSLog(@"Fetched comments: %@", comments);
} failure:^(NSError *error) {
NSLog(@"Failed: %@", [error localizedDescription]);
}];
}
List all comments in application
- (void)listCommentsByApplication {
[SZCommentUtils getCommentsByApplicationWithFirst:nil last:nil success:^(NSArray *comments) {
NSLog(@"Fetched comments: %@", comments);
} failure:^(NSError *error) {
NSLog(@"Failed: %@", [error localizedDescription]);
}];
}
Responding to changes in comment state¶
You can choose to be notified when comments are created
- (void)didCreate:(NSNotification*)notification {
NSArray *comments = [[notification userInfo] objectForKey:kSZCreatedObjectsKey];
id<SZComment> comment = [comments lastObject];
if ([comment conformsToProtocol:@protocol(SZComment)]) {
NSLog(@"Commented on %@", comment.entity);
}
}
- (void)respondToComments {
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(didCreate:) name:SZDidCreateObjectsNotification object:nil];
}