Sharing¶
Introduction¶
Socialize provides a complete set of helper functions to make sharing content in your app as easy as possible.
UI Elements¶
Share Dialog¶
The simplest way to allow users to share an entity (your content) is via the share dialog. Note that if you need to set meta information on the entity, you must do so with a separate call to [SZEntityUtils addEntity:success:failure:]
- (void)showShareDialog {
id<SZEntity> entity = [SZEntity entityWithKey:@"some_entity" name:@"Some Entity"];
[SZShareUtils showShareDialogWithViewController:self entity:entity completion:^(NSArray *shares) {
// `shares` is a list of all shares created during the lifetime of the share dialog
NSLog(@"Created %d shares: %@", [shares count], shares);
} cancellation:^{
NSLog(@"Share creation cancelled");
}];
}
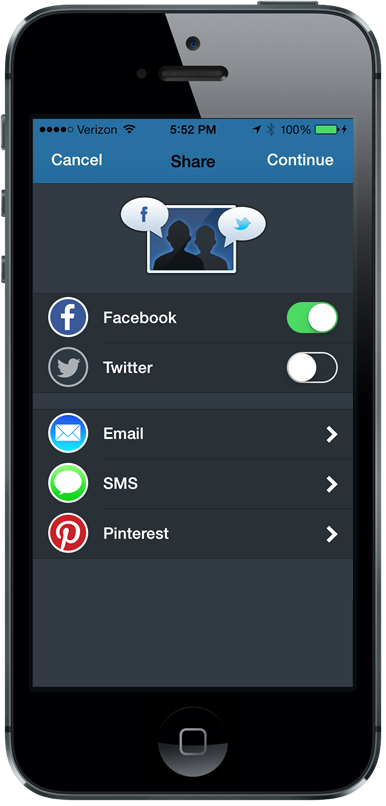
Custom Share Dialog Behavior¶
If you’d like, you can override some of Socialize’s share functionality
- (void)showShareDialogWithOptions {
id<SZEntity> entity = [SZEntity entityWithKey:@"some_entity" name:@"Some Entity"];
SZShareOptions *options = [SZShareUtils userShareOptions];
options.dontShareLocation = YES;
options.willShowSMSComposerBlock = ^(SZSMSShareData *smsData) {
NSLog(@"Sharing SMS");
};
options.willShowEmailComposerBlock = ^(SZEmailShareData *emailData) {
NSLog(@"Sharing Email");
};
options.willRedirectToPinterestBlock = ^(SZPinterestShareData *pinData)
{
NSLog(@"Sharing pin");
};
options.willAttemptPostingToSocialNetworkBlock = ^(SZSocialNetwork network, SZSocialNetworkPostData *postData) {
if (network == SZSocialNetworkTwitter) {
NSString *entityURL = [[postData.propagationInfo objectForKey:@"twitter"] objectForKey:@"entity_url"];
NSString *displayName = [postData.entity displayName];
SZShareOptions *shareOptions = (SZShareOptions*)postData.options;
NSString *text = shareOptions.text;
NSString *customStatus = [NSString stringWithFormat:@"%@ / Custom status for %@ with url %@", text, displayName, entityURL];
[postData.params setObject:customStatus forKey:@"status"];
} else if (network == SZSocialNetworkFacebook) {
NSString *entityURL = [[postData.propagationInfo objectForKey:@"facebook"] objectForKey:@"entity_url"];
NSString *displayName = [postData.entity displayName];
NSString *customMessage = [NSString stringWithFormat:@"Custom status for %@ ", displayName];
[postData.params setObject:customMessage forKey:@"message"];
[postData.params setObject:entityURL forKey:@"link"];
[postData.params setObject:@"A caption" forKey:@"caption"];
[postData.params setObject:@"Custom Name" forKey:@"name"];
[postData.params setObject:@"A Site" forKey:@"description"];
}
NSLog(@"Posting to %d", network);
};
options.didSucceedPostingToSocialNetworkBlock = ^(SZSocialNetwork network, id result) {
NSLog(@"Posted %@ to %d", result, network);
};
options.didFailPostingToSocialNetworkBlock = ^(SZSocialNetwork network, NSError *error) {
if (network == SZSocialNetworkFacebook) {
NSLog(@"%@", [SZFacebookUtils userMessageForError:error]);
//Example output: @"This app doesn't have permission to do this. To change permissions, try logging into the app again.”;
NSLog(@"%@", [SZFacebookUtils userTitleForError:error]);
} else {
NSLog(@"Failed posting to %d", network);
}
};
[SZShareUtils showShareDialogWithViewController:self options:options entity:entity completion:^(NSArray *shares) {
// `shares` is a list of all shares created during the lifetime of the share dialog
NSLog(@"Created %d shares: %@", [shares count], shares);
} cancellation:^{
NSLog(@"Share creation cancelled");
}];
}
Refer to Customizing the Content of Facebook Posts and Directly Opening an SMS or Email Composer for more details on customizing Facebook and SMS functionality
Manually instantiating the share dialog controller¶
If you need to, you can directly instantiate the share dialog and manage its lifecycle on your own.
- (void)manuallyShowShareDialog {
SZEntity *entity = [SZEntity entityWithKey:@"key" name:@"name"];
SZShareDialogViewController *share = [[SZShareDialogViewController alloc] initWithEntity:entity];
// share.title = @"A Custom Title";
// UIView *headerView = [[UIView alloc] initWithFrame:CGRectMake(0, 0, 320, 40)];
// headerView.backgroundColor = [UIColor greenColor];
// share.headerView = headerView;
//
// SZShareOptions *options = [SZShareUtils userShareOptions];
// options.text = @"Some default share text";
// share.shareOptions = options;
// share.dontShowComposer = YES;
share.completionBlock = ^(NSArray *shares) {
// Dismiss however you want here
[self dismissViewControllerAnimated:NO completion:nil];
};
// You can hide individual share types
// share.hideEmail = YES;
// share.hideSMS = YES;
// share.hideFacebook = YES;
// share.hideTwitter = YES;
// share.hidePinterest = YES;
// Present however you want here
[self presentViewController:share animated:NO completion:nil];
}
Working with Shares¶
Programmatic Sharing¶
Should you need to create a share programatically, you can do so. If you specify nil for the share options, the default share options from the user profile will be used.
- (void)createShare {
id<SZEntity> entity = [SZEntity entityWithKey:@"some_entity" name:@"Some Entity"];
SZShareOptions *options = [SZShareUtils userShareOptions];
// Optionally disable location sharing
// options.dontShareLocation = YES;
// Optionally add an image (Twitter only)
// options.image = [UIImage imageNamed:@"Smiley.png"];
[SZShareUtils shareViaSocialNetworksWithEntity:entity networks:SZAvailableSocialNetworks() options:options success:^(id<SZShare> share) {
NSLog(@"Created share: %@", share);
} failure:^(NSError *error) {
NSLog(@"Error creating share: %@", [error localizedDescription]);
}];
}
Customizing the Content of Facebook Posts¶
Before your share is sent off to Facebook, you are given an opportunity to modify the parameters used to post to the user’s wall
- (void)customizeFacebook {
id<SZEntity> entity = [SZEntity entityWithKey:@"some_entity" name:@"Some Entity"];
SZShareOptions *options = [SZShareUtils userShareOptions];
// http://developers.facebook.com/docs/reference/api/link/
options.willAttemptPostingToSocialNetworkBlock = ^(SZSocialNetwork network, SZSocialNetworkPostData *postData) {
if (network == SZSocialNetworkFacebook) {
[postData.params setObject:@"Hey check out this site i found" forKey:@"message"];
[postData.params setObject:@"http://www.facebook.com" forKey:@"link"];
[postData.params setObject:@"A caption" forKey:@"caption"];
[postData.params setObject:@"Facebook" forKey:@"name"];
[postData.params setObject:@"A Site" forKey:@"description"];
}
};
[SZShareUtils shareViaSocialNetworksWithEntity:entity networks:SZAvailableSocialNetworks() options:options success:^(id<SZShare> share) {
NSLog(@"Created share: %@", share);
} failure:^(NSError *error) {
NSLog(@"Error creating share: %@", [error localizedDescription]);
}];
}
Directly Opening an SMS or Email Composer¶
If you’d like to skip the share dialog and just present an email or SMS share dialog, you can do so
- (void)showSMS {
id<SZEntity> entity = [SZEntity entityWithKey:@"some_entity" name:@"Some Entity"];
SZShareOptions *options = [[SZShareOptions alloc] init];
options.willShowSMSComposerBlock = ^(SZSMSShareData *smsData) {
NSString *appURL = [smsData.propagationInfo objectForKey:@"application_url"];
NSString *entityURL = [smsData.propagationInfo objectForKey:@"entity_url"];
id<SZEntity> entity = smsData.share.entity;
NSString *appName = smsData.share.application.name;
smsData.body = [NSString stringWithFormat:@"Hark! (%@/%@, shared from the excellent %@ (%@))", entity.name, entityURL, appName, appURL];
};
[SZShareUtils shareViaSMSWithViewController:self options:options entity:entity success:^(id<SZShare> share) {
NSLog(@"Created share: %@", share);
} failure:^(NSError *error) {
NSLog(@"Error creating share: %@", [error localizedDescription]);
}];
}
- (void)showEmail {
id<SZEntity> entity = [SZEntity entityWithKey:@"some_entity" name:@"Some Entity"];
SZShareOptions *options = [[SZShareOptions alloc] init];
options.willShowEmailComposerBlock = ^(SZEmailShareData *emailData) {
emailData.subject = @"What's up?";
NSString *appURL = [emailData.propagationInfo objectForKey:@"application_url"];
NSString *entityURL = [emailData.propagationInfo objectForKey:@"entity_url"];
id<SZEntity> entity = emailData.share.entity;
NSString *appName = emailData.share.application.name;
emailData.messageBody = [NSString stringWithFormat:@"Hark! (%@/%@, shared from the excellent %@ (%@))", entity.name, entityURL, appName, appURL];
};
[SZShareUtils shareViaEmailWithViewController:self options:options entity:entity success:^(id<SZShare> share) {
NSLog(@"Created share: %@", share);
} failure:^(NSError *error) {
NSLog(@"Error creating share: %@", [error localizedDescription]);
}];
}
You can retrieve information about what has been shared in your application.
- (void)getSharesForEntity {
id<SZEntity> entity = [SZEntity entityWithKey:@"some_entity" name:@"Some Entity"];
[SZShareUtils getSharesWithEntity:entity first:nil last:nil success:^(NSArray *shares) {
NSLog(@"SHARES!!! %@", shares);
} failure:^(NSError *error) {
NSLog(@"Aww.. %@", [error localizedDescription]);
}];
}
- (void)getSharesById {
NSArray *ids = [NSArray arrayWithObject:[NSNumber numberWithInteger:10]];
[SZShareUtils getSharesWithIds:ids success:^(NSArray *shares) {
NSLog(@"SHARES!!! %@", shares);
} failure:^(NSError *error) {
NSLog(@"Aww.. %@", [error localizedDescription]);
}];
}
- (void)getSharesByUser {
[SZShareUtils getSharesWithUser:nil first:nil last:nil success:^(NSArray *shares) {
NSLog(@"SHARES!!! %@", shares);
} failure:^(NSError *error) {
NSLog(@"Aww.. %@", [error localizedDescription]);
}];
}
- (void)getSharesByUserAndEntity {
id<SZEntity> entity = [SZEntity entityWithKey:@"some_entity" name:@"Some Entity"];
[SZShareUtils getSharesWithUser:nil entity:entity first:nil last:nil success:^(NSArray *shares) {
NSLog(@"SHARES!!! %@", shares);
} failure:^(NSError *error) {
NSLog(@"Aww.. %@", [error localizedDescription]);
}];
}
List all shares in the application