Users¶
Introduction¶
Every device running Socialize has a “User”, however Socialize automatically manages this User for you so there is no need to explicitly create one.
A User has both Settings and Activity. The Settings correspond to the User’s preferences while using Socialize whereas the Activity relates to their social actions.
User UI Elements¶
User Profile¶
You can display a profile view for any user which includes their recent activity
- (void)showUserProfile {
// Pass nil to show the current user
[SZUserUtils showUserProfileInViewController:self user:nil completion:^(id<SZFullUser> user) {
NSLog(@"Done showing profile");
}];
}
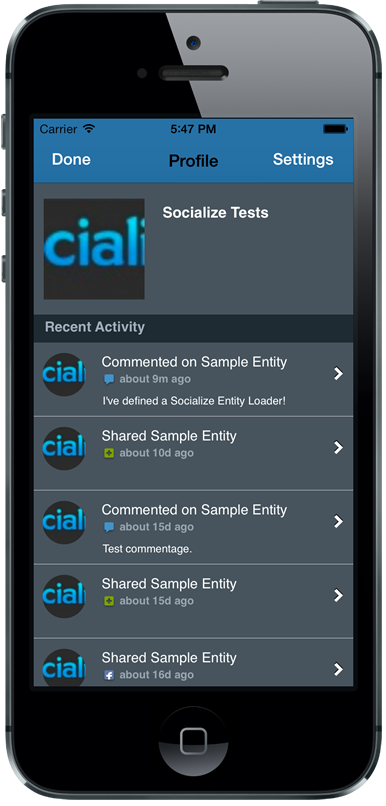
User Settings¶
To allow a user to update their settings you can simply present them with the User Settings view:
- (void)showUserSettings {
[SZUserUtils showUserSettingsInViewController:self completion:^{
NSLog(@"Done showing settings");
}];
}
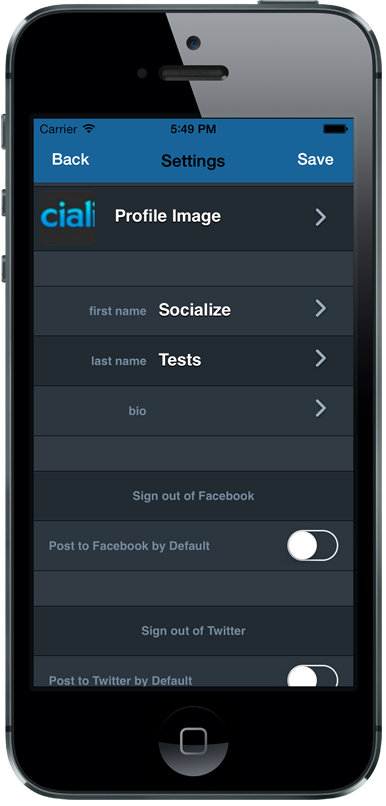
You can manually show the settings for further customization
- (void)manuallyShowUserSettings {
SZUserSettingsViewController *settings = [[SZUserSettingsViewController alloc] init];
// Uncomment to hide logout buttons
// settings.hideLogoutButtons = YES;
settings.completionBlock = ^(BOOL didSave, id<SZFullUser> user) {
// Dismiss however you want here
[self dismissViewControllerAnimated:NO completion:nil];
};
// Present however you want here
[self presentViewController:settings animated:NO completion:nil];
}
Link Dialog¶
If you’d like to prompt the user to link to a third party on your own, you can do so:
- (void)showLinkDialog {
if (![SZUserUtils userIsLinked]) {
[SZUserUtils showLinkDialogWithViewController:self completion:^(SZSocialNetwork selectedNetwork) {
NSLog(@"Linked!");
} cancellation:^{
NSLog(@"Not linked!");
}];
}
}
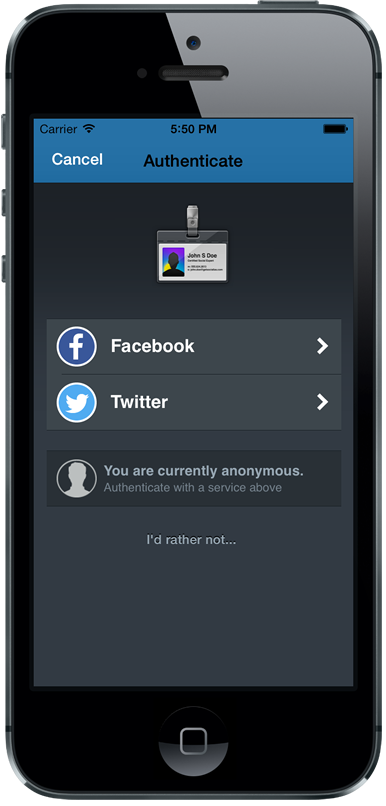
Working with Users¶
Getting a User¶
To obtain a reference to the current user simply call the getCurrentUser method
- (void)getCurrentUser {
id<SZFullUser> currentUser = [SZUserUtils currentUser];
NSLog(@"My name is %@ %@!", [currentUser firstName], [currentUser lastName]);
}
To obtain a User object users other than the current user
- (void)getOtherUser {
// I have stashed a list of ids somewhere
NSArray *ids = [NSArray arrayWithObjects:[NSNumber numberWithInteger:1], [NSNumber numberWithInteger:2], [NSNumber numberWithInteger:3], nil];
[SZUserUtils getUsersWithIds:ids success:^(NSArray *users) {
NSLog(@"Got users: %@", users);
} failure:^(NSError *error) {
NSLog(@"Failed to get users: %@", [error localizedDescription]);
}];
}
If you want to build your own UI to update a User’s settings you can simply call the saveUserSettings method
- (void)saveSettings {
// Specify a new image and description for the current user
SZUserSettings *settings = [SZUserUtils currentUserSettings];
UIImage *newImage = [UIImage imageNamed:@"Smiley.png"];
settings.profileImage = newImage;
settings.bio = @"I do some stuff from time to time";
// Update the server
[SZUserUtils saveUserSettings:settings success:^(SZUserSettings *settings, id<SocializeFullUser> updatedUser) {
NSLog(@"Saved user %d", [updatedUser objectID]);
} failure:^(NSError *error) {
NSLog(@"Broke: %@", [error localizedDescription]);
}];
}
User Activity¶
SZActionUtils provides for retrieval of the activity stream for a given user.